If you’re a programmer or just getting started with coding, you might have come across the term “Scanline C.” It’s common in graphics programming and other code-intensive projects. Ending Scanline C properly can be tricky for beginners, but don’t worry. This guide will explain everything you need to know about how to end Scanline C in a way that’s simple, error-free, and beginner-friendly.
What Does It Mean to End Scanline C?
Ending Scanline C simply means completing a specific operation in your program where a scanline—a row of pixels or a section of graphical data—is processed or drawn to a display. In computer graphics, scanlines are integral to rendering an image or completing a frame.
To end Scanline C, you need to close the operation correctly so that your program can move on to the next step without glitches. If it’s not done properly, it could lead to errors, crashes, or a broken output. For example, if you’re rendering an image on the screen, a mistake in ending Scanline C can result in distorted visuals or incomplete graphics.
Why Do You Need to End Scanline C?
Ending Scanline C is important for several reasons:
- To Ensure Program Stability: If Scanline C isn’t closed correctly, your program might throw errors, crash, or freeze.
- To Prevent Visual Errors: Especially in graphics programming, not ending Scanline C properly could lead to corrupted or incomplete images.
- To Optimize Performance: Properly ending this process ensures smoother execution, reducing the chances of lag or unnecessary processing loops.
- To Avoid Debugging Headaches Later: If you skip this step or do it wrong, finding and fixing errors in your code can be time-consuming.
In short, ending Scanline C ensures your code runs smoothly and produces the expected results.
Steps to End Scanline C Without Errors
Ending Scanline C may seem challenging at first, but by following a systematic approach, you can do it correctly. Here are the key steps to ensure you finish Scanline C without any hiccups:
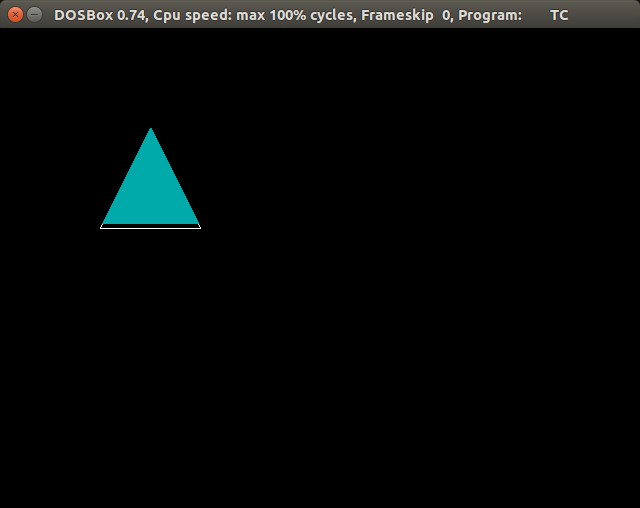
Check Your Code for Mistakes
Before ending Scanline C, double-check your existing code. Ensure you’ve written the proper logic to process each scanline. Look for missing brackets, incomplete loops, or misplaced semicolons that could interfere with how Scanline C is processed.
- Verify your variables and data structures.
- Confirm that any loops controlling the scanlines (e.g., for or while) are set up correctly.
Add the Correct Syntax
Ending Scanline C often requires adding specific syntax to finalize the operation. Depending on the programming language or framework you’re using, this might involve:
- Calling a function to close the scanline operation (e.g., EndScanline();).
- Adding termination characters like ; or } in the correct places.
- Ensuring the scanline data is properly saved or stored before moving to the next operation.
For example, in C-based programming, you might use something like this:
c
CopyEdit
void EndScanline() {
// Code to close the scanline process
// Ensure all pixel data is stored
}
Test Your Program to Be Sure
Once you’ve added the necessary syntax and fixed any errors, test your program to ensure everything works as expected.
- Run your program: Look for any graphical glitches or crashes that indicate an issue with Scanline C.
- Check for warnings or errors: Your compiler or IDE should point out any major problems in your code.
- Debug if needed: Use debugging tools to step through your code and see how it handles Scanline C.
Common Mistakes When Ending Scanline C
Even experienced programmers sometimes make mistakes when ending Scanline C. Here are some of the most common errors to avoid:
- Forgetting to Close the Scanline: Not adding the necessary syntax or function to end the scanline operation is a common oversight.
- Misplacing Brackets or Parentheses: Missing or misplaced {} or () can disrupt the program’s flow, especially in loops.
- Using the Wrong Functions: Calling the wrong function or using an incorrect parameter can result in incomplete scanline operations.
- Skipping Error Checking: Not testing your program after making changes can lead to unnoticed errors.
- Overwriting Scanline Data: Accidentally overwriting data for one scanline before finishing it can cause glitches.
By keeping these pitfalls in mind, you can save yourself a lot of time and frustration.
Quick Fixes for Scanline C Problems
If you encounter issues while trying to end Scanline C, here are a few quick fixes to try:
- Revisit Your Code Logic: Check if you’ve missed any essential steps or made an error in your syntax.
- Look for Missing Semicolons or Braces: These small mistakes can often cause big problems.
- Use a Debugger: Debugging tools can help you pinpoint exactly where your code is going wrong.
- Consult Documentation: Refer to the programming language or framework’s official documentation for guidance.
- Seek Help Online: If you’re stuck, forums like Stack Overflow or GitHub can provide helpful solutions.
Using Tools to Debug Your Code
Debugging tools can be a lifesaver when working with complex programs or unfamiliar code. Here’s how you can use these tools effectively to debug Scanline C:
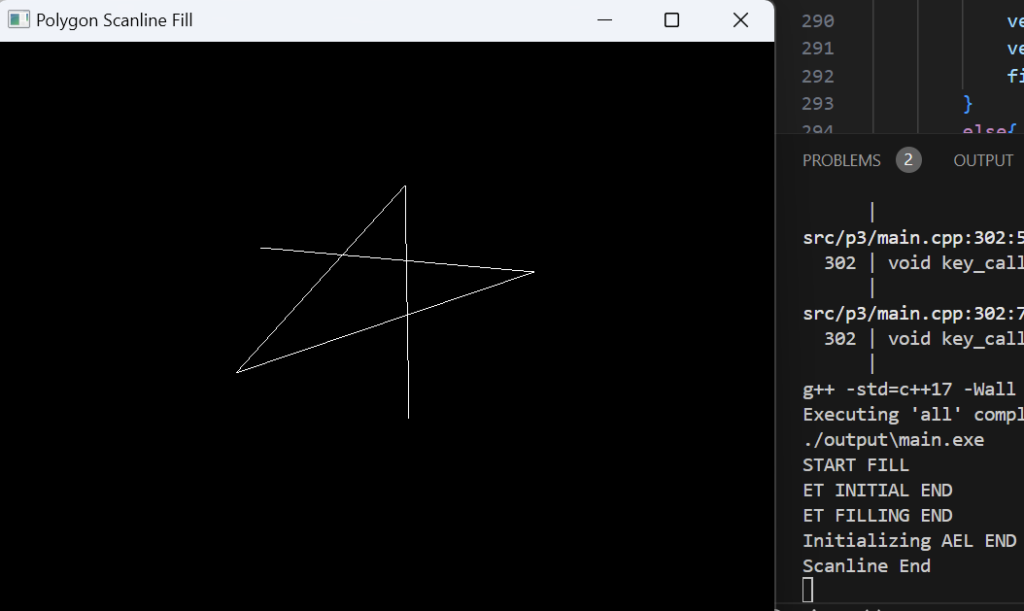
Finding the Right Solution for Your Program
Choose a debugger that works well with your programming environment. For example:
- GDB (GNU Debugger): Ideal for debugging C programs.
- Visual Studio Debugger: A powerful tool for Windows developers.
- Online Debugging Tools: If you’re coding in a browser-based IDE, use its built-in debugger.
Double-Checking Your Scanline Syntax
Use debugging tools to step through your code line by line. This allows you to identify:
- Any skipped steps in the Scanline C process.
- Errors caused by incorrect syntax or misplaced loops.
By analyzing your code carefully, you can quickly fix errors and improve the overall performance of your program.
Real-Life Example of Ending Scanline C
Let’s say you’re building a simple game in C that involves drawing pixel art. Each frame is composed of multiple scanlines. To end Scanline C correctly, you might use the following code snippet:
c
CopyEdit
for (int y = 0; y < SCREEN_HEIGHT; y++) {
for (int x = 0; x < SCREEN_WIDTH; x++) {
DrawPixel(x, y, color); // Render each pixel
}
EndScanline(); // Finalize the scanline
}
In this example, EndScanline(); ensures that all the data for the current row of pixels is processed before moving to the next row. Without this step, the frame might render incorrectly or fail to display.
The Bottom Line
Ending Scanline C may seem complicated at first, but with the right approach, it becomes much easier to manage. Always double-check your code, use the correct syntax, and test thoroughly to ensure everything runs smoothly. By avoiding common mistakes and leveraging debugging tools, you can effectively end Scanline C without errors.
Whether you’re working on a graphics project or just learning to code, mastering this process will save you time, reduce frustration, and help you produce high-quality results. So, keep practicing, and you’ll soon become a pro at handling Scanline C like a seasoned developer!